Need Help With API Testing?
When using your favorite mobile apps or online resources, you are interacting with the application programming interface (API) behind the scenes without even knowing it.
Without delving into how the provided functions are implemented, the API serves as an interface between two software applications, allowing them to communicate with one another according to the specified rules. If you want to know the current weather, you can embed a small weather widget on your homepage, which will send a rules-defined request to the API of some weather service and receive a rules-defined response containing a data parcel.
Here’s an even simpler example: take the waiter as the API of the restaurant. At the restaurant, you place an order based on the courses defined on the menu. The waiter takes your order, and that’s where your participation ends. You’re not interested in what happens next except for getting your meal.
All popular software products have an open API part. You have a document with a list of what and how you can ask and what you will get in response. You can interact with the API from a web page, with special tools, or directly from the code. At the same time, more APIs necessitate additional testing to ensure they meet various requirements. With this testing, an API may function as planned.
We at IntelliSoft realize the importance of API testing. When we cooperated with one of our clients, an industrial equipment manufacturer, we incorporated IoT water heating sensors. We fine-tuned them for the client’s end-users so they could manage their systems remotely. We received an API for water heat sensors from the client’s team, which we incorporated using JavaScript. Moreover, by the use of REST API, we were able to connect the client’s accounting software to their ERP system.
We are ready to share our experience, describing the app and tools for testing REST API and methods in below.
Table of Contents
What Is API Testing?
API testing is a type of testing, which involves testing the API directly, as well as within integration testing, to check whether the API meets expectations in terms of functionality, reliability, performance, and security of the application. In API testing, the main focus will be on the business logic level of the software architecture.
Business logic analysis and the security of application data are the main objectives of API testing. An API test is typically carried out by submitting requests to one or more API endpoints and contrasting the results with what is anticipated.
Since APIs serve as the principal interface to app functionality and GUI tests are challenging to maintain Agile software development and DevOps methodologies’ short release cycles and rapid changes, experts see API testing as essential to test automation.
API in Terms of the 3-Tier Architecture
The three components of a software architecture are the client, the server, and the database. The client typically takes the form of a web browser or a mobile app, which displays the requested data, while the server is responsible for implementing the actual business logic. Transfer protocol-based requests are how clients and servers exchange information. The API defines the structure and reasoning behind requests relying on a particular protocol.
In REST, the most common way for software to transfer data is when clients send HTTP/HTTPS requests to the server. The server gets the requested data from the database, changes it based on the business logic, and sends it back to the client in JSON, XML, or another format as a response. UI shows it to the user at the end.
SOAP API, another kind of web API, is a web communication technology that has been around for a while yet is still widely used today. In addition to the HTTP/HTTPS protocols, SOAP supports TCP, SMTP, and FTP. However, it can only read and write XML.
Types of API Methods
API methods are a crucial aspect of how applications interact with each other through APIs (Application Programming Interfaces). There are different types of API methods used to determine the kind of request being made to an API endpoint. Here are the main types:
- GET: This method is used to retrieve data from the server. It is a read-only operation and does not modify any data on the server.
- POST: POST is used to send data to the server to create a new resource. It is often used for submitting forms or uploading files.
- PUT: PUT is used to update or replace an existing resource on the server. When you use PUT, you typically send the entire resource, including any unchanged data.
- DELETE: As the name suggests, DELETE is used to request the removal of a resource from the server.
These API methods are part of the HTTP protocol and are widely used in RESTful APIs. However, there are other methods beyond these basic ones, such as PATCH, HEAD, OPTIONS, and more, each with its own specific use case.
The choice of API method depends on the action you want to perform on the server, whether it’s retrieving data, creating new records, updating existing data, or deleting resources. Understanding these methods is essential for developers working with APIs to ensure proper communication and data manipulation.
Types of Output of an API
An output of API may consist of the following three elements:
- Any type of data
There ought to be a function in the API that can add together two integer numbers.
Long add(int a, int b)
The numbers themselves must be provided as one of the input parameters. The output should be the sum of two numbers that are both integers. This output needs to be checked against what can happen next.
There is a need to make calls, such as to:
add (1234, 5656)
- Status (say Pass or Fail)
Say you have the following function:
Lock()
Unlock()
Delete()
As an output, it provides any value, such as True (in the event that the operation was successful) or false (in the event that an error occurred).
A more precise description of a test case would say it’s possible to call functions in any of the scripts and afterwards check for changes in either the database or the app’s graphical user interface (UI).
- Call another API function
In this particular scenario, we invoke one of the API functions, which then causes another function to be invoked.
For instance, the First API function can be called to delete a particular record from the table, and the Second API function can be called to REFRESH the database. Both functions are called by the First API function.
REST API Testing Types
Quality assurance (QA) engineers approach API testing from a variety of perspectives. They examine whether it gives proper outputs in the appropriate format, sends responses in an acceptable amount of time, and how well it connects with software for the presentation layer. The responses of an API to edge cases (such as failures, unexpected or extreme inputs, etc.) and potential security threats are evaluated by testers. Thus, let’s examine each of these facets.
- Unit testing
Developers usually write unit tests. Sometimes, this team is busy with development and there is no agreement about covering the code with unit tests. Unit testing is a good practice for reducing the technical debt and the cost of maintaining the system in the future. Implementing this approach, however, is often a matter of available resources. Atomicity and isolation of API methods allow covering the code by tests well.
Now, let’s compare API testing and unit testing side by side.
Validation testing
Unlike unit tests, which examine small chunks of functionality, validation tests are at a higher level and answer a set of questions after detecting which software can move on to the next stage of development.
Validation questions may sound as follows:
- Product-specific questions: “Is this the functionality that was needed?”
- Expected behavior questions: “Does this method behave as expected?”
- Performance-related questions: “Is this method using resources as independently and efficiently as possible?”
API performance tests
QA engineers test the operation and performance of an API by intentionally making or simulating API calls to confirm that the API can manage the expected load or a higher load than predicted.
Security testing
Safety testing checks the type of authentication and data encryption used using HTTP. Authentication, permissions, and access controls are all part of this examination, in particular:
- What kind of authentication is needed to use the API
- How well the system encrypted sensitive data
- What permission checks are set for resource access, etc.
Security testing, known as PEN and penetration testing, must focus on multiple factors. Experts must consider the access points of APIs, the data flow, and any shadow APIs that are no longer in use but are still operational.
API integration and reliability tests
The primary concern of the first one is the flow of information between APIs, ensuring that they are well-connected and do not introduce errors into other APIs.
In the second case, developers should check an API for any potential disconnections after synchronization with multiple devices.
Load testing
Increasing the number of users should not affect application performance. Load testing will identify performance and availability issues with scaling services and check API performance under normal conditions.
Load testing is a resource-intensive process. Often, experts do it only once before handing over the product’s next version at the customer’s or product owner’s request. This exam ensures that the APIs do not contain memory leaks or similar flaws that could create problems after lengthy operations.
Runtime error detection
Runtime error detection aims to provide a thorough check that corrections have been implemented following a near-final evaluation of the known flaws and issues from earlier tests. In contrast to other API testing methods, Runtime error detection is solely concerned with the API’s actual behavior. This test analyzes the results of implementing the API, paying particular attention to execution faults, testing the system’s error-handling capabilities, and monitoring memory leaks.
Interoperability testing
This one is a type of testing that applies to SOAP APIs. Functional interoperability between SOAP APIs is tested by ensuring compliance to web service interoperability profiles. WS-compliance is tested to ensure that standards such as WS-Addressing, WS-Discovery, WS-Federation, WS-Policy, WS-Security, and WS-Trust are properly implemented and used.
Fuzz tests
This test is necessary to check the API by forcing incorrect data into the system to try to force a crash. This kind of “worst-case scenario” lets engineers check the API to its very limits.
Throughout each of the last three tests, enable runtime error detection. With this technology, our APIs are able to report any errors that occur while they are in operation.
Difference between Manual Testing & Automated Testing
Automated testing requires expensive setup and maintenance. After all, if the application flow changes, your API call sequence or parameters may be wrong. Any modification like this involves adjustments in the test automation framework, which may be expensive to put up. This expenditure is usually worth it if you run a suite of tests daily or with every test environment update.For instance, experts recommend automating something if it requires at least ten tests.
You want to know whether a build contains too many faults at that time before we even begin testing because a new build will be required after the errors are fixed. Moreover, API test automation offers several benefits. Because you can fast-build test scenarios, you can readily make test cases for many edge cases. Also, you may incorporate your build workflows and run the tests less frequently manually.
Anyway, there are benefits and drawbacks to both types of examinations. Testing by hand takes a long time and is tiresome. Yet, its greatest power is its superiority in dealing with complex situations. Nonetheless, its speed and breadth of coverage are both substantial improvements. Coding and test upkeep are essential for automated testing.
What is REST Assured API Testing?
Rest Assured is a Java library tailored for the testing of RESTful web services. It excels in verifying both XML and JSON-based web services. This versatile tool accommodates a wide spectrum of HTTP request methods, such as GET, POST, PUT, PATCH, DELETE, OPTIONS, and HEAD. Its primary utility lies in its ability to validate and confirm the responses generated by these requests.
This library has gained popularity in the software testing community and is integrated with popular frameworks like JUnit and TestNG for efficient test case creation and execution. It simplifies the process of ensuring that your RESTful APIs function correctly and deliver the expected results. You can use it to confirm that your web services respond as intended and to catch any errors or discrepancies in their output. Rest Assured is a valuable asset for maintaining the reliability and integrity of your RESTful web services.
How to Test API
The API layer of any application is one of the most important software components of the system. It is the conduit that connects the client to the server (or one microservice to another), manages business processes, and presents services that benefit users. If it fails, it puts not just the app, but the whole chain of business processes built around it, at risk. API testing has many other benefits besides preventing one from this risk.
Thus, let’s have a look at how to test API step-by-step.
Step 1: Come up with API testing requirements
At the heart of this stage is the identification of the types of testing you want to perform to improve the quality of the software and eliminate all possible defects.
Positive testing scenarios help verify the basic functionality of the IT product, while negative tests verify whether the system can handle security and performance issues.
In particular, at this stage, decide on:
- Testing aspects
- Testing priorities
- Goals of the API
- Target audience
- APIs it interacts with
- App’s workflow
- Features and functionality
- Issues under observation
- Desired outcomes
- The definition of Pass and Fail.
Step 2: Establish the API examination environment
Configuring the input and output parameters of the database and server helps shape the proper environment for API testing.
To improve the accuracy of the tests, it is worth giving preference to simulating the real-time conditions required for public use of the software. This practice helps verify the quality of the application by identifying critical issues that prevent the software from functioning correctly, including performance issues.
Choosing the optimal toolkit helps achieve a more efficient testing process. The variety of tools available satisfies a wide range of business needs. The tools support an API architecture, help create detailed reporting, and adapt to different types of testing, such as security and performance.
Step 3: Make a trial API call
Make a quick call to the API before digging into further in-depth testing to ensure that everything is working properly and that nothing has been broken.
Step 4: Identify the input parameters
Prepare for every conceivable combination of inputs. You are going to use them in your test cases, and then later – for the authentication of the results, in order to evaluate whether or not the API functions as planned.
Step 5: Develop API test cases
Once you are ready with all the preparations, you can write and run test cases. In conclusion, you will evaluate the actual outcomes versus the anticipated ones. It is a good practice to group them according to the category of the test. The following are some examples of API test cases:
- Testing how the API works in the system when it doesn’t get a response.
- Monitoring any disruptions or events caused by the output.
- Testing value in light of the given parameters: you are responsible for defining the input and verifying the authenticity of the result, which can be any kind of information or status (such as Pass or Fail).
- Validating resources affected by the API call and verifying the effect of data structure alterations on the system.
How to Select Tools for API Testing
Many API testing tools exist, so you may wonder how to pick a suitable toolkit.
Defect categories
Where do the most severe bugs manifest, and what kinds of bugs are we talking about here? You can find the answer to that question on a bug tracker in less time than it takes to eat lunch. Bugs in the application’s business logic, data storage layer, or user interface can be the focus of such analysis (GUI).
Looking For QA Specialists?
Test automation of the business logic via unit tests won’t be of much use if the more critical issues are in the user interface. That won’t be the jumping off point at all. Still, come back to this point after picking the tool.
Team fit
Who will do the automating? If programmers or testers will be doing the automation, the tool should probably be a code library or package. In the same way, a group of testers who don’t know much about technology will feel more at ease if the tool has a record/playback front end.
Some tools record actions and then turn them into code; others create a visual front end that lets programmers “drop in” to see the code behind the visualization.
The main problem is that specialists who are supposed to learn how to use the tool need to be willing, able, and have time to do so. If the testing process is behind, giving testers a new tool to learn will add more work and slow down the software delivery process to the customer.
Automation, especially up front, will slow down testing even more if the regression-test process takes days or weeks to run, leading to a backlog until testers reach some break-even threshold. Engineers will still need to deal with the existing backlog when the tool is no longer a bottleneck.
Programming language & development environment
If the tool supports a programming language, you have two options: you can either write in the same language as the production programmers or choose an exceptionally powerful high-level language like Ruby that is simple to learn.
It’s possible to fail the commit and force the developers to repair the bug if the test is in the same language as the production code and runs during the CI run. An even better scenario would be if the tool could function as a plug-in within the developer’s IDE, reducing the amount of context switching required.
It’s doubtful that programmers will learn a new tool or put in the time to maintain the tool when it detects “failures” if the tool operates outside of the IDE and uses a different programming language.
Installation & test-data management process
Tools that suit you would probably offer ways to create accounts, clear orders, export an account and its orders as “known good test data,” and then re-import them. These activities would let audits always start with a set-up. That would speed up the whole testing process for everyone.
An additional frequent target for enhancement is the provision of test servers on demand by a branch or commit. For the most part, this activity is critical if a team is pursuing CI and wants end-to-end checking to run as part of the CI.
The proper functional test tool may automate test setup if it’s the main bottleneck in the testing process or if it’s necessary for achieving repeatable testing.
Version control and CI
Most teams who wish to avoid automation delays incorporate the tool into the CI process. In other words, the CI system verifies the code, produces a build, executes the unit tests, and generates an actual server (if necessary) and a client, possibly installing the software on a mobile device. The CI system then initiates the execution of end-to-end tests using the functional tool.
Sending data from the tool to the CI system is necessary for its utilization. The tool must operate from the command line and generate output that the CI system can understand. Or, it must be feasible to capture the output and turn it into a format that the CI system can read. Numerous CI systems have attractive dashboards and pie charts for displaying results to stakeholders.
How Much Will Your Project Cost?
Once the tool runs under CI, the real power lies in getting it to report failures to the programmer responsible for them. Thus, it’s critical to determine who made the commit that failed, and then work with the programmer to either correct the code or debug the test to mark it as passed.
Reports
A test tool is useless if it does not produce actionable results. Unless the team intends to shift the info into another system with superior reports, dashboards and charts can be powerful features.
Tracking test runs over time can be a powerful feature as well. The degree of a stakeholder determines the types of outcomes they care about. High-level executives may care less about pass/fail percentages and more about overall trends. Upper-level managers are interested in learning about the process’s overall structure. Experts will want to see a video of the test run to get a better sense of what went wrong.
Supported platforms & tagging
Creating a page object with typical function characteristics is a frequent technique for end-to-end tools. Most platforms will have comparable use cases, such as login, product page display, tag, search, checkout, etc. The automated checks use these functions. A path-to-purchase check can be reused on every device since page objects are created at runtime; in other words, you can perform the same test against a new page object.
Some features don’t overlap; they’re exclusive to the product’s full-sized web version.
You may keep tabs to which tests refer in specific browsers by labeling them. If the test needs a specific kind of page object, one option is to register those objects so that you can check for their presence.
With tags, you may tell the tool to “perform all tests for the edge browser full size,” or only the tests that use a specific API, or just the tests that check the profile, and so on.
Related readings:
- Making Sense of Databases: How to Choose the Right One
- Migrating Legacy Systems: Essential Stages and Tips from Pros
- What Threatens Your Web Application Security
- What Is Software Testing and When Your Product Needs It?
- RabbitMQ vs Kafka: Choosing the Right Messaging System for Your Needs
Top 10 API Testing Tools
Our team has analyzed a lot of tools for testing REST API before coming up with the best options for you.
ReadyAPI
With ReadyAPI, you can quickly test the functionality, load, and security of SOAP, RESTful, GraphQL, and many other web services in the CI/CD pipeline. It has a single interface and helps DevOps and Agile teams speed up API quality. With just a few clicks, teams can easily make tests that are based on data and add security scans. ReadyAPI also lets you virtualize web services like SOAP, RESTful, TCP, etc., so that testing pipelines don’t have to depend on them.
A quick and easy way to get insights and metrics from an interactive dashboard is one of the most demanded features of ReadyAPI. Besides, the solution provides detailed reports and analytics in a number of different formats, such as HTML, CSV, and so on. You can make your own data or bring in data from other places and share it.
ReadyAPI offers three modules, the API Test Module, the API Performance Module, and the API Virtualization Module, each of which is priced at $759 USD per license, $5,639 USD per license, and $1,059 USD per license, respectively. They are billed annually and trial periods last 14 days.
ACCELQ
ACCELQ is a vendor that does API testing using no-code and the cloud to automate API testing. Its unique attribute is no code test automation.
ACCELQ gives users a place to build API tests without having to write complicated syntax. This can work well for QA teams that don’t know a lot about programming. ACCELQ Tests can be automated for element identification, data definition, and test case generation.
In general, ACCELQ is free. However you may also try team (manual and automated testing) for $50/month billed monthly or $40/month yearly.
Katalon Platform
Katalon Studio is yet another free API testing tool for Web, Desktop, and Mobile apps. It simplifies development by providing a unified environment for all necessary plugins and frameworks. The two different modes, Manual and Scripting, make it accessible to users of all skill levels.
Katalon Studio offers testers with low to medium programming skills a good UI/UX. Users can use functions like drag and drop, built-in keywords, and searching and choosing test cases for repetition with ease. For seasoned automation testers, using these features can take a lot of time.
Katalon is compatible with both SOAP and RESTful APIs. Both automated and exploratory testing can benefit from its utilization. The tool includes CI/CD support and provides a comprehensive solution and structure.
Katalon Studio’s starting monthly price is $54.0. Katalon Studio offers two distinct plans, Runtime Engine for monthly $54.00 and Studio Enterprise available for $79 per month.
Postman
Postman began as a Chrome plugin and then evolved into an on-premise solution for both Mac and Windows. The basic features to note include an intuitive REST client and a rich user interface. This tool is accessible for both exploratory and automated testing.
Users can submit all of the requests and anticipated responses to their coworkers in one package, making it easy for them to share their knowledge with the team.
Postman employs new advanced choices in version 10.6.0 to assist users in intelligently organizing collections and API pieces (mock servers, monitors, tests, and documentation) created from API schemas. You can test your APIs with various data points by using data files. If there are any test failures or issues, you can arrange the scheduled run to send email notifications.
Free to $12 per user each month is the price of this product.
REST-Assured
Testing REST services in the Java domain is simple using REST-Assured. The tool is open-source. REST-Assured accepts requests and responses in XML and JSON.
REST-Assured offers a few built-in features and supports Given/When/Then BDD syntax. You don’t have to be an expert in HTTP to utilize the program. The tool interacts well with the Serenity automation framework without a hitch.
Because REST-Assured is a Java library, connecting it with Junit, TestNG, or any other Java framework is simple, and integrating it with CI/CD is also relatively quick. Given-when-then notation, which is very similar to if-else note, ensures that humans can read the code hassle-free as it is in the English language.
REST-Assured, an open-source and free-to-use test framework licensed under Apache 2.0, intends to bring the simplicity of using various languages to the Java sector.
Apigee
Apigee is a management solution for API gateways useful for transporting data between cloud applications and services. It provides a proxy layer in front of services, hence hiding the backend service APIs.
Apigee consists of two key components. Secondly, there are the Apigee services, which enable the creation, deployment, and management of API proxies. The second component is the Apigee runtime, through which all API communication is routing and processing. The API monitoring is powered by artificial intelligence, so it’s possible to recognize any faults with a few clicks.
The API proxy keeps sensitive data secure by acting as a buffer between the app developer and your backend service. You can make changes to the service implementation as long as the public API stays the same. Existing client apps will continue to function with minimal disruption in the event of backend modifications if the API at the front end remains consistent.
The starting package costs $300 monthly, while a pro-pack can get as high as $2250 per month.
SoapUI
SoapUI is an API-focused headless functional testing tool. Easy testing of RESTful and SOAP-based APIs and Web Services is possible. The simple Drag-and-drop, Point-and-click interface makes it easy to create tests. Users of the Free plan can get their hands on the complete code so they may implement the things they like most.
The fact that it supports both SOAP APIs and REST services is one of the key elements contributing to this tool’s popularity. Together with this, SoapUI’s ReadyAPI offers end-to-end testing for frequently used protocols including GraphQL, Java Message Service (JMS), Java Database Connectivity (JDBC), and other APIs.
The newest version of SoapUI supports GraphQL APIs and adds other enhancements that make API testing more efficient. To improve testing security, SoapUI now disables the Load and Save project scripts by default.
SoapUI has both a paid version called Pro and a free version called Open Source. ReadyAPI has three modules: API Test Module, API Performance Module, and API Virtualization. Each module costs $759 USD per license, $5,639 USD per license, and $1,060 USD per license, respectively. They have 14-day trial periods and are billed once a year.
Assertible
Assertible is widely one of the best API testing tools due to its emphasis on dependability and its support for API tests throughout the entire pipeline, beginning with continuous integration and ending with delivery.
As if that weren’t enough, Assertible has some fantastic tools for its users. It can be connected to Slack, Zapier, and GitHub. Assertible also checks the validity of assertions made in response to HTTP requests.
Assertible has introduced a feature known as Encrypted variables. This feature provides a new method for storing tokens, passwords, and secret data fields required by tests, hence enhancing API testing security procedures. Encrypted variables are not only easy to use, but are also based on a cryptographically sound mechanism for secure storage.
This tool for testing REST APIs comes in three packages. The standard version costs $25 per month, while the startup plan costs $50 per month. The business plan, which has the most features, costs $100 per month and is the most expensive package. Also, there is a free personal plan that lets you run API tests easier to handle and learn how Assertible works.
JMeter
While being designed for load testing, JMeter is good for functional API testing. This software supports reviewing test results. The tool works with CSV files automatically, enabling the team to swiftly generate distinct parameter values for the API tests.
Because JMeter and Jenkins are integrated, users can use the API tests in CI workflows. It is also good to test the performance of static and dynamic resources.
Its primary focus is on web applications, but the tool is also suitable for measuring the most fundamental aspects of performance. JMeter is compatible with a wide variety of servers and protocols, including HTTP, HTTPS, SOAP, LDAP, FTP, and others. It is free to use and uses an open-source code base. JMeter, at long last, boasts a user interface that is both interactive and simple to operate.
Insomnia
To help you get some shut-eye, Kong offers the free Insomnia tool. It’s comparable to Postman in a way both can test APIs. Insomnia’s installer is included, which is similar to Postman’s. You may get the installer and begin testing immediately.
Like Postman, Insomnia requires little to no training time and has several functionalities that are immediately apparent to the user. Insomnia allows for both manual and automated API testing, with the ability to specify assertions if a given test case should succeed or fail.
Insomnia permits the creation of new custom plugins to enhance your testing framework. Insomnia is capable of generating code snippets based on the API schemas.
Individual plan costs just $5 per month; team will have to pay $12 monthly; finally, enterprise costs $25.
Best Practices for API Testing
Are you interested in more tips and tricks for API testing?
It is crucial that you properly organize your test cases by their intended purpose as this will facilitate easier upkeep and provide a more comprehensive picture of the application’s current state.
Make sure all stakeholders are involved in the design of these requirements, and cover the API’s workflow, how it fits in the application, and any edge circumstances.
To test API requests, you require a testing environment that closely mimics production. While testing against stubs and drivers might help launch or hasten development, we must always do end-to-end tests in a sandbox environment.
Pay close attention to the testing triangle. In other words, you automate what can’t be tested using unit or integration tests. Automation may significantly reduce costs and, when used appropriately, can identify critical problems at an early stage.
Challenges of API Testing
Testing procedures that are exhaustive not only help quality assurance and development teams produce code that functions, but also produce code that functions well. When testing application programming interfaces (APIs), the following six problems are particularly important.
Leaving Out Response Time Assertions
Developers can program API exams to look for a wide variety of conditions, including particular status codes and the contents of responses. How good are those parts if an API request takes ten seconds to answer when verifying method correctness? Response time assertions are a simple yet crucial part of any API test, although testers frequently disregard them.
Establish response time assertions that are rational and reflect the time interval that you or your developers believe it should take. If you begin with a high threshold, it will be simple to go down and determine what aspects of the request are successful. An expression of a high threshold for reaction time is much preferable than having none at all.
Testing APIs in a Vacuum
API testing can be done by one person, but as soon as a test is in your workflow, you need to bring in the right teams in case there are any problems. API tests don’t always fail for the same reasons, and when they do, it can affect a lot of different people. So, when tests fail, different teams in your organization need to pay attention. If you set up test failure notifications to only go to you, you’re adding time, work, and headaches to your workflow.
As soon as an API test is added to your operational or development process, notify the appropriate parties using their preferred notification methods. By integrating your API tests with Slack, PagerDuty, Jenkins, and other technologies, you can enable your entire team to quickly resolve API testing obstacles. This practice will add notifications for the team in charge of resolving API issues.
Building Irrelevant Tests
One of the most difficult aspects of API testing is building tests that don’t simulate the user experience. It is simple to put up tests that validate independent services and endpoints, and then to call it a day once they all pass. Inventory API testing, check; shopping cart API testing, check; etc.
It’s likely that your end-users would utilize these approaches in tandem, not alone. In the short run, building tests without thinking how APIs will be consumed may be faster. In doing so, however, you will not test across concerns, which could prevent you from discovering and troubleshooting potentially critical API flaws.
Integration tests are a type of classical software test that span many functional units or modules. There may be less work involved than you anticipate in creating integration tests for APIs.
Not Validating Data
You might receive a notification in the event that API tests are completed successfully. On the other hand, if the responses from your APIs do not contain the correct data, then the test might as well have been considered unsuccessful.
It is necessary to check that your API is returning the correct data in order to handle this significant difficulty associated with API testing. Teams can describe what a successful API call looks like by checking for criteria such as specified status codes, HTTP headers, and JSON or XML properties. Testing can then be performed in accordance with this definition.
Not Including API Dependencies
Failure to integrate API dependencies in your API testing plan is a significant concern. Conventional software integration testing involves testing distinct code modules together to ensure that they function consistently and reliably. It is usual for current programs that extensively rely on web services to rely on web services that reside outside of your four walls. Thus, testing only your own APIs does not provide a comprehensive view of how your application will function in the actual world.
Your API is a product that depends on partner services, and if any of those services fail, your API could also fail your customers. As a general rule, if your product depends on a service, you should test and keep an eye on it. Third-party integrations can be just as valuable as your own APIs, and if your app or service is broken, your users won’t know (or care) whose service is failing.
Managing API Tests Manually
There is no reason to continue manually developing API tests when there are so many development tools available to assist in automating your workflow. When done manually, these tasks can be time-consuming and distracting. You can start monitoring your APIs from the beginning of development all the way through production using API testing tools we recalled in this post.
Now, let’s take a look at IT process automation tools.
What Are IT Automation Software Solutions?
Automation software solutions are essential applications used in the field of Information Technology (IT) to streamline and simplify various tasks, reducing manual intervention and improving efficiency. These IT process automation software are designed to automate repetitive and routine processes, allowing IT professionals to focus on more strategic and complex tasks.
List of IT Automation Software Tools
Here is a list of IT process automation software as of 2023:
- ActiveBatch: A comprehensive IT automation tool that streamlines workflows and processes.
- Redwood RunMyJobs: Offers robust automation capabilities for managing complex IT tasks.
- Tidal Automation: Provides automation solutions for workload management and scheduling.
- NinjaOne: A versatile automation platform suitable for IT management tasks.
- Atera: Focuses on IT service management and automation for MSPs (Managed Service Providers).
- Jira Service Management: Known for its IT service desk automation capabilities.
- ManageEngine Endpoint Central: Offers IT asset management and automation solutions.
- SysAid: An IT service management and automation platform designed for efficiency.
- BMC Control-M: Provides comprehensive workload automation for enterprises.
- Broadcom CA Automic: Known for its IT automation and orchestration capabilities.
- Broadcom CA IT Process Automation Manager: Focuses on streamlining IT processes through automation.
- SMA OpCon: Offers automation solutions for IT operations and workload automation.
API Testing in a Nutshell
If you haven’t done either before, you might believe API testing is more difficult than UI test automation, but you’d be wrong. Difficulties often happen when specialists rush through too many ideas without first laying a solid foundation.
When you understand how clients communicate with servers using the HTTP protocol, it is much simpler to compose automation scripts for API testing. It’s essential to have a solid understanding of these principles in order to develop effective test cases for API services.
IntelliSoft’s quality assurance and testing experts can create test scripts that perform more than just API testing. Our professionals eliminate the need for teams to switch between different tools to conduct end-to-end testing of their APIs. In addition to the traditional performance and load testing, users can now perform their functional tests through API testing and monitoring. Whenever you need to integrate API testing and best practices, we’re here to lend a helping hand.
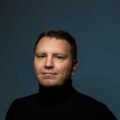
AboutKosta Mitrofanskiy
I have 25 years of hands-on experience in the IT and software development industry. During this period, I helped 50+ companies to gain a technological edge across different industries. I can help you with dedicated teams, hiring stand-alone developers, developing a product design and MVP for your healthcare, logistics, or IoT projects. If you have questions concerning our cooperation or need an NDA to sign, contact info@intellisoftware.net.